ChatGPT 4oとは
「ChatGPT 4o」は「チャットジーピーティーフォーオー」と読むそうです。
GPT-4oは、GPT-4レベルのインテリジェンスを提供する最新のフラッグシップモデルで、テキスト、音声、視覚の機能が大幅に向上しているとのこと。
詳しくは以下の公式アナウンスをチェックしてみてください。
Introducing GPT-4o and more tools to ChatGPT free users
誰が使えるの?無料でも使えそう。
ChatGPT Plus と Team ユーザーへの展開を開始しており、エンタープライズ ユーザー向けの提供も近日中に開始される予定とのこと。
ChatGPT Freeへの展開を開始し、利用制限を設けています。Plus ユーザーには、無料ユーザーの最大 5 倍のメッセージ制限があり、Team ユーザーと Enterprise ユーザーにはさらに高い制限があります。
いつまでのデータを元にしているのですか?と質問した際の回答
私のトレーニングデータは2023年9月までの情報に基づいています。それ以降の出来事や新しい情報については知識がありません。そのため、最新のニュースやトピックについては、他の最新の情報源を参照する必要があります。
会話もスムーズ
動画内でスマホアプリのChatGPT4oと会話してみたが、めちゃくちゃスムーズに会話ができた。
楽しいっ!
画像生成速度はそんなに変わらなかった。
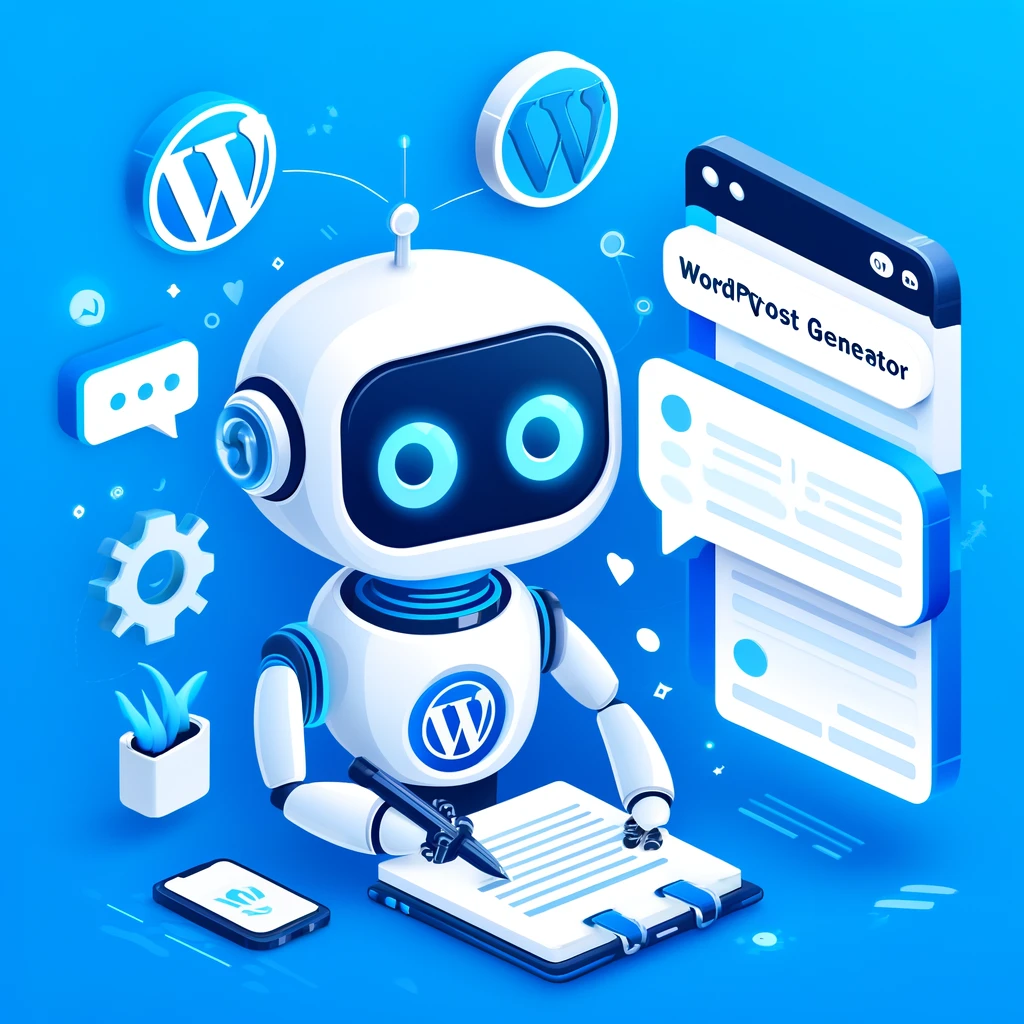
WordPressの記事をChatGPTで自動生成させ、投稿させるプラグインを作らせてみた。
以下の指示を元に、ChatGPT 4oにプログラムをさせてみた。
WordPressのプラグインを作って欲しい。
プラグインの仕様
・ChatGPTに本文、タイトルを考えさせる。
・テキストボックスにいれたキーワードを元に本文やタイトルを考える。
・ボタンを押して自動で投稿させる。
詳細は動画を参照してみて欲しいが、非常に高速かつ間違いの少なそうなプログラムをしていることがわかる。
生成したプラグインのコード
動作確認したが多分動いてそう。
もっと詳細な指示を出していけばさらに高度なことはできるはず。
回答
WordPressのプラグインを作成するためのコードを提供します。このプラグインは、ChatGPT APIを使用してキーワードに基づいた投稿のタイトルと本文を生成し、自動的に投稿する機能を持っています。以下は、プラグインの基本的な構成です。
- プラグインのファイル構成
my-chatgpt-plugin.php: プラグインのメインファイル - my-chatgpt-plugin.php
- my-chatgpt-plugin.js
- ChatGPT APIキーの取得
このプラグインを動作させるには、OpenAIのChatGPT APIキーが必要です。OpenAIの公式サイトでAPIキーを取得し、my-chatgpt-plugin.php
ファイルの$chatgpt_api_key
変数に設定してください。
インストール手順
my-chatgpt-plugin.php
とmy-chatgpt-plugin.js
をWordPressのプラグインフォルダにアップロードします(例:wp-content/plugins/my-chatgpt-plugin/
)。
WordPress管理画面にログインし、プラグインを有効化します。
管理メニューに「ChatGPT Post Generator」が表示されるので、そこからキーワードを入力して投稿を生成できます。
このプラグインは基本的な機能を実装していますが、さらにカスタマイズして使いやすくすることも可能です。
コード
my-chatgpt-plugin.php
<?php /* Plugin Name: My ChatGPT Plugin Description: A plugin to generate post title and content using ChatGPT based on a keyword. Version: 1.0 Author: Your Name */ if (!defined('ABSPATH')) { exit; } class MyChatGPTPlugin { public function __construct() { add_action('admin_menu', array($this, 'create_plugin_settings_page')); add_action('admin_init', array($this, 'setup_sections')); add_action('admin_init', array($this, 'setup_fields')); add_action('admin_enqueue_scripts', array($this, 'enqueue_scripts')); } public function create_plugin_settings_page() { $page_title = 'ChatGPT Post Generator'; $menu_title = 'ChatGPT Post Generator'; $capability = 'manage_options'; $slug = 'chatgpt_post_generator'; $callback = array($this, 'plugin_settings_page_content'); add_menu_page($page_title, $menu_title, $capability, $slug, $callback); } public function plugin_settings_page_content() { ?> <div class="wrap"> <h1>ChatGPT Post Generator</h1> <form method="post" action="options.php"> <?php settings_fields('chatgpt_post_generator'); do_settings_sections('chatgpt_post_generator'); submit_button(); ?> </form> </div> <?php } public function setup_sections() { add_settings_section('first_section', 'Generate Post', array(), 'chatgpt_post_generator'); } public function setup_fields() { add_settings_field('keyword', 'Keyword', array($this, 'field_callback'), 'chatgpt_post_generator', 'first_section', ['label_for' => 'keyword']); register_setting('chatgpt_post_generator', 'keyword'); } public function field_callback($arguments) { ?> <input type="text" name="keyword" id="keyword" value="<?php echo get_option('keyword'); ?>" /> <button type="button" id="generate_post_button" class="button button-primary">Generate Post</button> <?php } public function enqueue_scripts() { wp_enqueue_script('my_chatgpt_plugin_script', plugin_dir_url(__FILE__) . 'my-chatgpt-plugin.js', array('jquery'), null, true); wp_localize_script('my_chatgpt_plugin_script', 'my_chatgpt_plugin', array('ajax_url' => admin_url('admin-ajax.php'))); } } new MyChatGPTPlugin(); // Handle AJAX request add_action('wp_ajax_generate_post', 'generate_post'); function generate_post() { if (isset($_POST['keyword'])) { $keyword = sanitize_text_field($_POST['keyword']); // Call ChatGPT API $chatgpt_api_key = 'YOUR_CHATGPT_API_KEY'; $api_url = 'https://api.openai.com/v1/engines/davinci-codex/completions'; $response = wp_remote_post($api_url, array( 'headers' => array( 'Authorization' => 'Bearer ' . $chatgpt_api_key, 'Content-Type' => 'application/json', ), 'body' => json_encode(array( 'prompt' => 'Generate a blog post title and content based on the keyword: ' . $keyword, 'max_tokens' => 300, )), )); if (is_wp_error($response)) { wp_send_json_error('API request failed.'); } $body = wp_remote_retrieve_body($response); $data = json_decode($body, true); if (isset($data['choices'][0]['text'])) { $generated_text = $data['choices'][0]['text']; $title = strtok($generated_text, "\n"); $content = substr($generated_text, strlen($title) + 1); // Create new post $post_data = array( 'post_title' => wp_strip_all_tags($title), 'post_content' => $content, 'post_status' => 'publish', 'post_author' => get_current_user_id(), ); $post_id = wp_insert_post($post_data); if ($post_id) { wp_send_json_success('Post created successfully.'); } else { wp_send_json_error('Failed to create post.'); } } else { wp_send_json_error('No text generated.'); } } else { wp_send_json_error('No keyword provided.'); } } ?>
my-chatgpt-plugin.js
jQuery(document).ready(function($) { $('#generate_post_button').on('click', function() { var keyword = $('#keyword').val(); if (keyword) { $.ajax({ url: my_chatgpt_plugin.ajax_url, method: 'POST', data: { action: 'generate_post', keyword: keyword, }, success: function(response) { if (response.success) { alert('Post created successfully.'); } else { alert('Failed to create post: ' + response.data); } }, error: function() { alert('An error occurred while generating the post.'); } }); } else { alert('Please enter a keyword.'); } }); });